¶ Google account login
¶ Preparation
Configure in Google API Console Credentials Console (opens new window) and Authing Management Console (opens new window).
¶ Integrate Google login steps
¶ Step 1: Add Google Login Component Dependency
Enter: https://github.com/Authing/authing-binary in the swift package search bar.
Authing-binary (opens new window) depends on Guard-iOS SDK (opens new window).
Select Up to Next Major Version 1.0.0 for the dependency rule.
Click Add Package and check Google.
¶ Step 2: Modify project configuration
Configure the Google Login component bounce URL:
- Select the Xcode project, click the plus sign in Targets -> Info -> URL Types.
- URL Schemes Add iOS URL scheme of Google console.
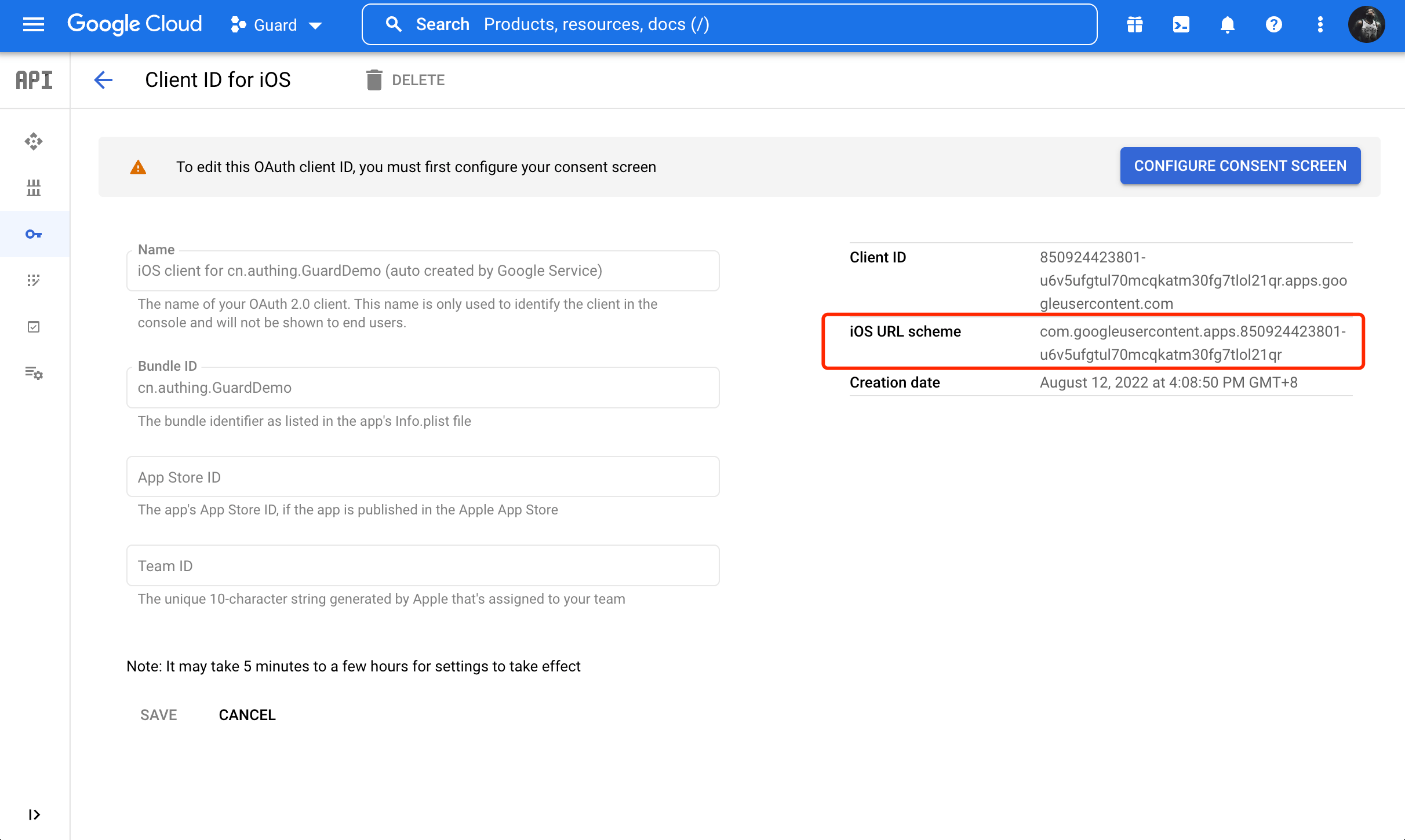
iOS URL schemes
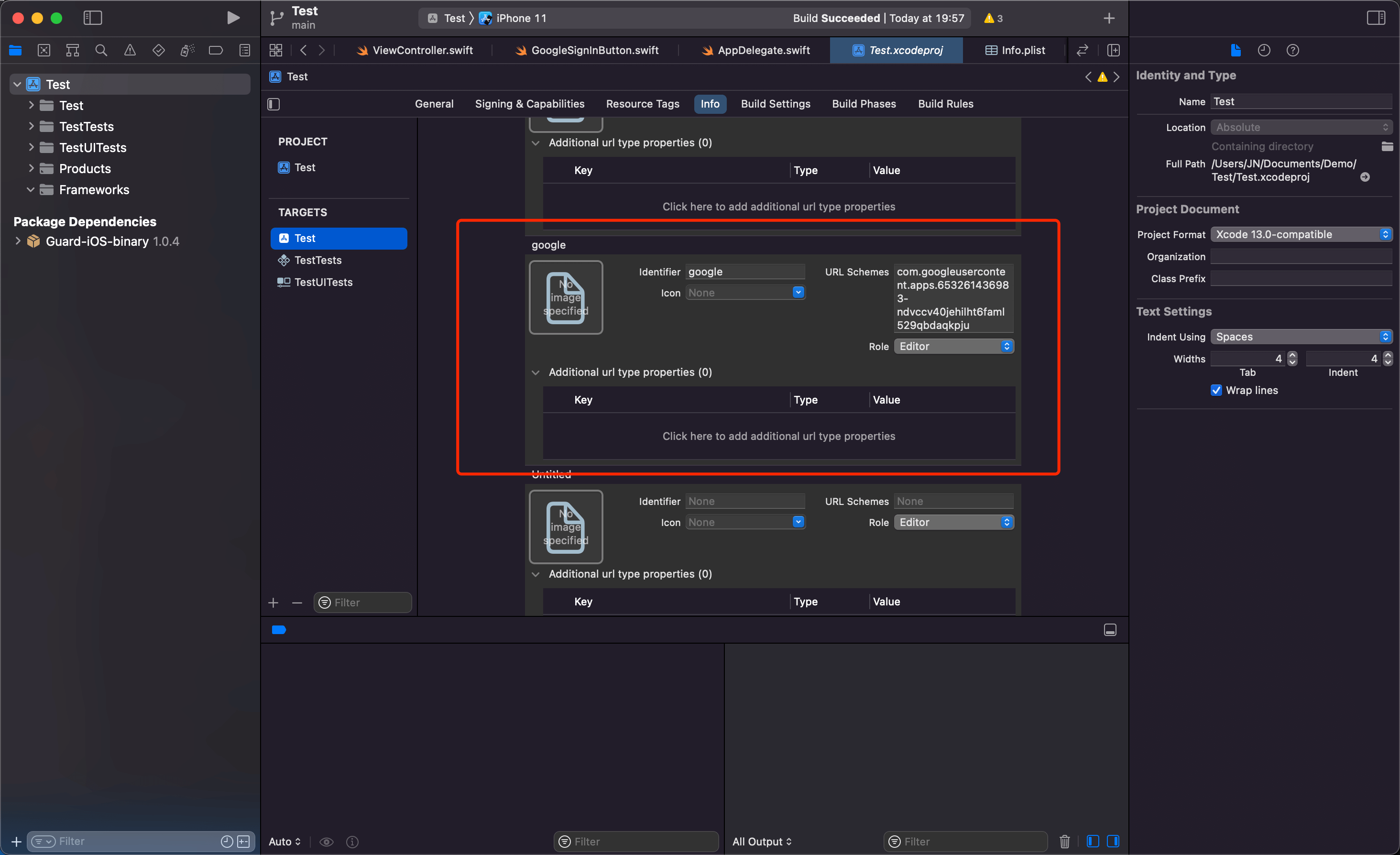
Add URL Types
¶ Step 3: Initialize Google components
Add import Guard and import Google to AppDelegate or SceneDelegate.
Call Authing.start() to initialize Guard SDK.
Google.register needs to pass in the clientID and serverClientId issued by the Google console.
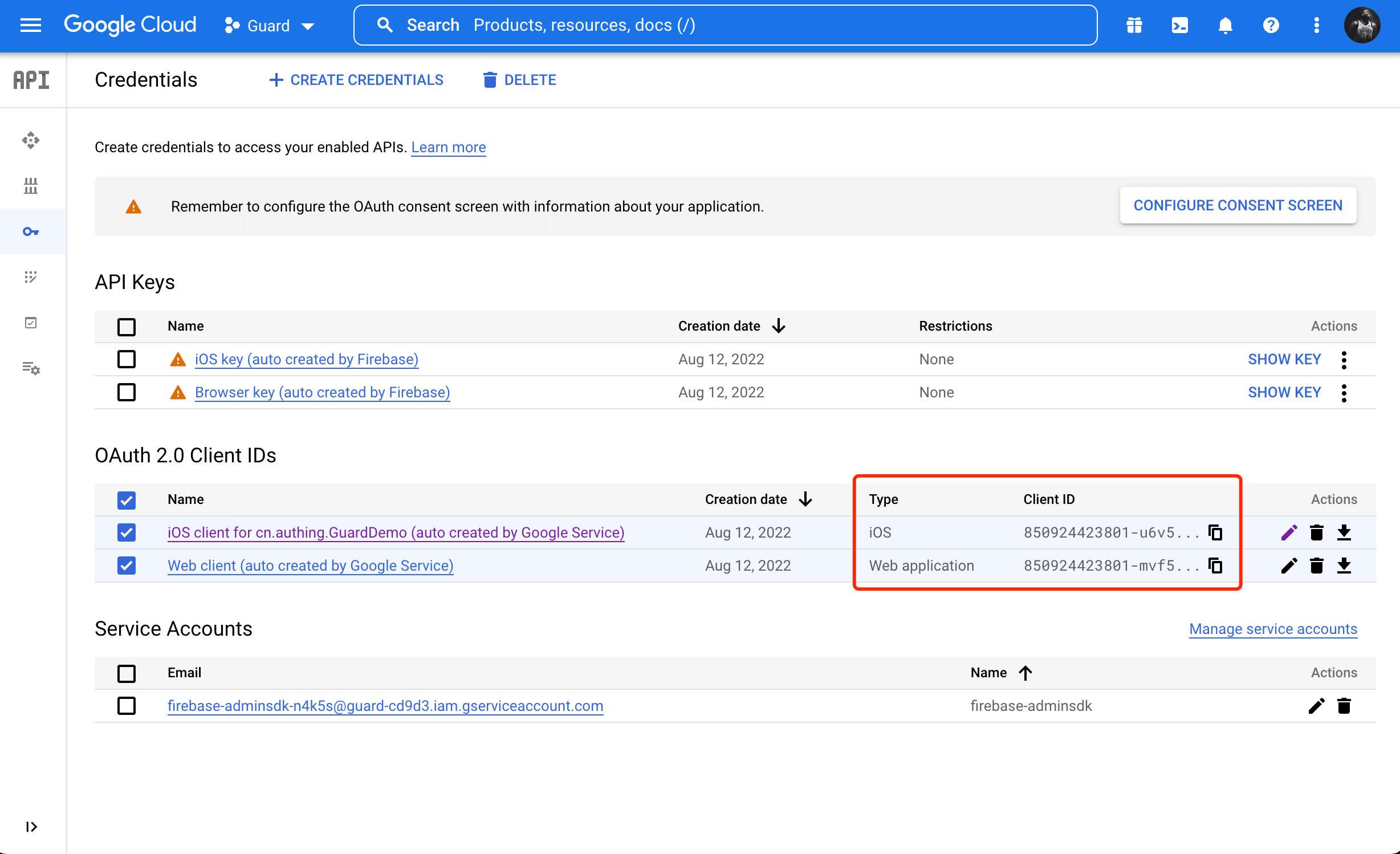
clientID is the ClientID of the Google Console iOS app, serverClientId is the ClientID of the Google Console web application.
import Guard
import Google
Authing.start(<#AUTHING_APP_ID#>)
Google.register(clientID: <#iOS ClientId#>, serverClientId: <#Oauth Web ClientId#>)
¶ Step 4: Add callbacks
After successfully logging in and returning to the application, if SceneDelegate is used, the following functions need to be overloaded in SceneDelegate.swift:
func scene(_ scene: UIScene, openURLContexts URLContexts: Set<UIOpenURLContext>) {
if let url = URLContexts.first?.url {
_ = Google. handleURL(url: url)
}
}
If SceneDelegate is not used, the following functions need to be overloaded in AppDelegate.
func application(_ app: UIApplication, open url: URL, options: [UIApplication. OpenURLOptionsKey : Any] = [:]) -> Bool {
return Google. handleURL(url: url)
}
¶ Step 5: Initiate Google Authorization
The Google Sign-in component provides three authorization methods:
- The developer calls the one-click login API when login is required:
Google.login(viewController: <#ViewController that hosts the view#>) { code, message, userInfo in
if (code == 200) {
// userInfo: user information
}
}
- With the semantic Hyper Component we provide, you only need to place one in the xib:
GoogleSignInButton
Set Module to Google, click GoogleSignInButton after Build success to log in.
- If you want to access the entire process of Google authorization yourself, after getting the authorization code, you can call the following API in exchange for Authing user information:
func loginByGoogle(_ code: String, completion: @escaping(Int, String?, UserInfo?) -> Void)
parameter
authCode
Google authorization code
example
AuthClient().loginByGoogle(authCode) { code, message, userInfo in
if (code == 200) {
// userInfo: user information
}
}